Unpacking Hidden MCP Gems: Transports
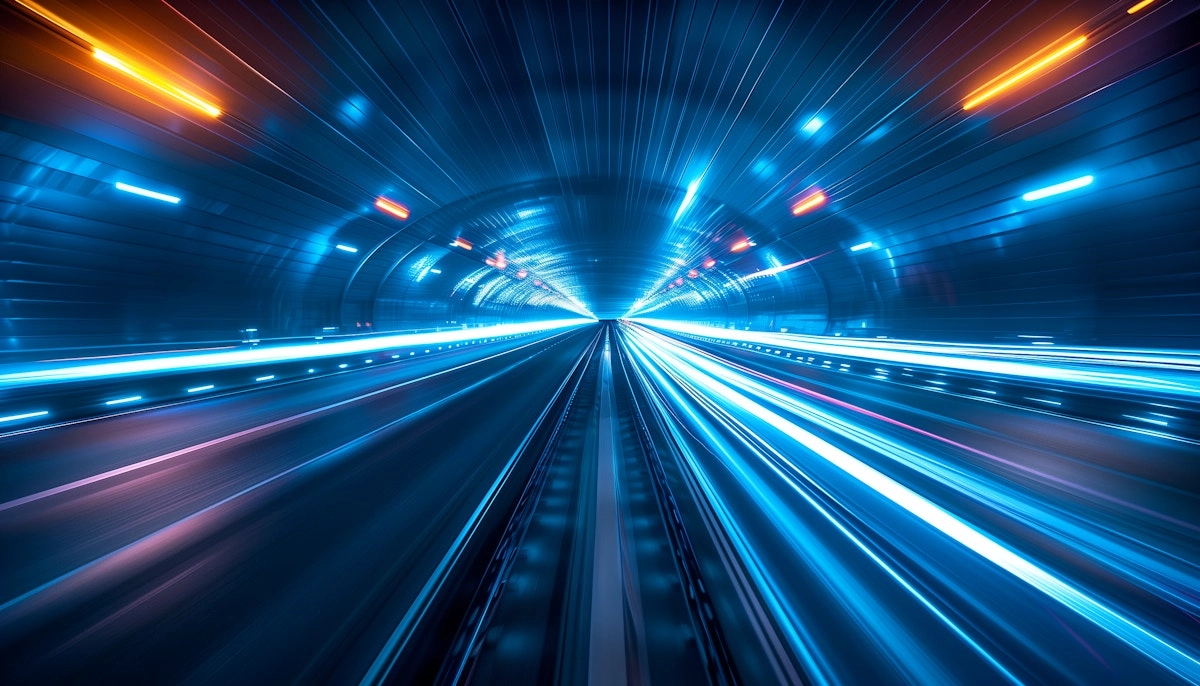
Transports are the backbone of MCP communication, responsible for converting MCP protocol messages to JSON-RPC 2.0 and back. By abstracting the message framing, they allow you to focus on business logic instead of low-level plumbing.
Built-in Transport Types
Standard I/O (STDIO)
Ideal for local CLI tools and simple process integrations, STDIO reads from standard in and writes to standard out, turning any subprocess into an MCP server or client with minimal setup.
Server-Sent Events (SSE)
SSE over HTTP enables persistent, one-way streaming from server-to-client and uses HTTP POST for client-to-server messages. It’s perfect for environments where only server-to-client updates are needed, but you must guard against DNS rebinding (i.e. validate origin headers).
Extending with Custom Transports
Beyond STDIO and SSE, MCP’s Transport interface makes it relatively trivial to plug in WebSockets, binary protocols (e.g. MessagePack), or even proprietary channels. You just need to make sure that your implementation conforms to the Transport interface:
interface Transport {start(): Promise<void>; // Start processing messagessend(message: JSONRPCMessage): Promise<void>; // Send a JSON-RPC messageclose(): Promise<void>; // Close the connectiononClose?: () => void; // Callbackonmessage?: (msg: JSONRPCMessage) => void; // Callbackonerror?: (err: Error) => void; // Callback}
Transport Tips & Tricks
Managing Connection Lifecycle & Resilience
- Graceful Startup & Teardown: Always sequence your transport’s `start()`, normal operation, and `close()` calls to avoid resource leaks. For example, on a client crash ensure you call `close()` or trigger your `onClose` callback to clean up any pending promises.
- Timeouts & Heartbeats: Define sensible timeouts for connecting, sending, and receiving messages. If a peer doesn’t respond within your timeout window, trigger reconnection logic or surface an error to the user.
- Reconnection Logic: On transient network failures, implement exponential-backoff retries rather than hammering the server. Exposing a configurable max retry count helps prevent infinite loops.
Data Integrity & Message Validation
- Schema Validation: Before processing, validate each JSON-RPC message against your schema.
- Size & Rate Limits: Enforce maximum message sizes to avoid out-of-memory or denial-of-service scenarios. Combine this with rate-limiting (requests per second) to guard against floods.
- Input Sanitization: Strip or escape any untrusted fields in params to prevent injection attacks, especially if you later pass data into command-line subprocesses or evaluate code.
Authentication & Authorization
- Pluggable Auth Layers: For HTTP-based transports (SSE, WebSockets, REST), integrate OAuth 2.0 or JWT validation; for STDIO, pull credentials from environment variables or config files as recommended .
- Least-Privilege Capabilities: Only grant each client the minimal set of MCP methods and resources it needs. Reject unauthorized calls early in your transport layer to avoid unnecessary server work.
- Secure Token Handling: Store tokens in memory only; avoid writing them to logs or disk. Rotate credentials periodically and support token revocation when possible.
Network & Transport Security
- TLS Everywhere: Encrypt all network transports with TLS (HTTPS, WSS). Even in “internal” environments, this thwarts man-in-the-middle attacks.
- Origin & Host Validation: For SSE or WebSocket servers, validate the Origin/Host headers on incoming connections. Never bind to 0.0.0.0 in production—use a specific interface or 127.0.0.1 to limit exposure.
- DNS Rebinding Mitigations: When using SSE, ensure your CORS policy and DNS settings cannot be subverted. Check that incoming DNS names resolve only to allowed IPs before accepting connections.
Observability & Monitoring
- Structured Logging: Emit JSON-structured logs for each transport event (connection open/close, send/receive, errors) so they can be ingested by observability platforms.
- Metrics & Health Checks: Track counters (messages sent/received, errors) and gauges (current connections, queue lengths). Expose a /health endpoint that runs a quick send/receive test on a loopback transport.
- Back-pressure Handling: If your transport buffers outbound messages, monitor the buffer length. Once it exceeds a threshold, either apply back-pressure to upstream producers or drop/expire old messages based on your application’s needs.